Detecting a specific character, like a double quote (“), in a string is a common task in JavaScript. This guide will cover several methods to check if a character is a double quote. It will help you choose the best one for your needs. If you’re validating input, parsing text, or handling data formats, these techniques can help. They will let you manage double quotes in JavaScript.
Why Check for Double Quotes in JavaScript?
Double quotes are often used in strings. They may affect how JavaScript interprets your code or processes input. You might want to verify if a certain character in a string is a double quote for reasons such as:
- Validating user input for security.
- Processing data from text fields.
- Handling JSON data, which often involves double-quoted keys and values.
Methods to Check for Double Quotes in JavaScript
Here are several methods to check if a character in a string is a double quote. Each approach has its advantages and specific use cases.
1. Using the === Operator
The simplest way to check if a character is a double quote is to compare it with the double quote character (“). Here’s how:
javascript
let character = ‘”‘;
if (character === ‘”‘) {
console.log(“The character is a double quote.”);
} else {
console.log(“The character is not a double quote.”);
}
Explanation:
- This code checks if the variable character holds a double quote by comparing it directly with “.
- If they are equal, it prints that the character is a double quote.
This method is straightforward and works best for single-character checks.
2. Checking for Double Quotes in a String Using indexOf()
If you want to check if a string contains a double quote somewhere within it, you can use the indexOf() method.
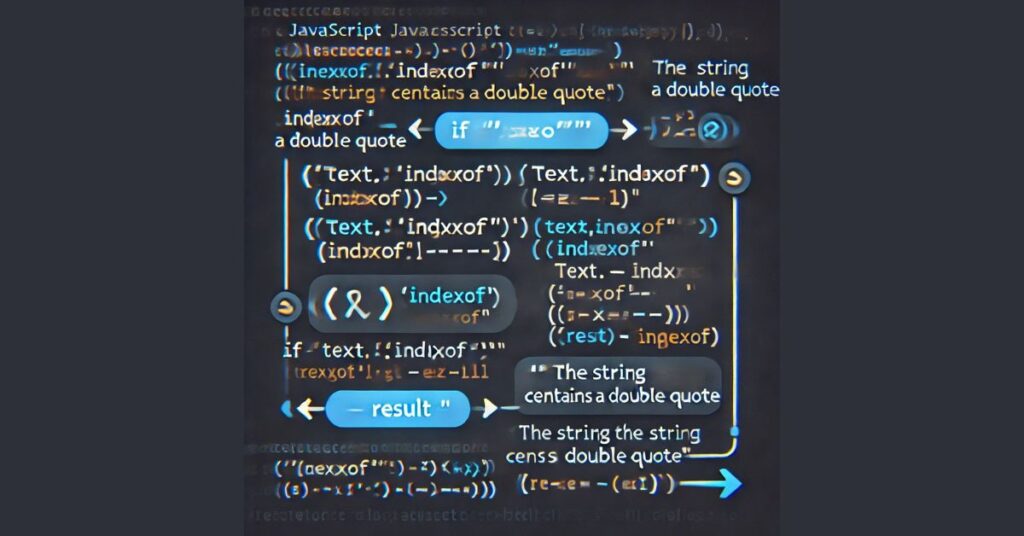
javascript
let text = ‘Hello “World”‘;
if (text.indexOf(‘”‘) !== -1) {
console.log(“The string contains a double quote.”);
} else {
console.log(“The string does not contain a double quote.”);
}
Explanation:
- indexOf(‘”‘) returns the position of the first occurrence of a double quote in the string. If it’s not found, indexOf() returns -1.
- This is useful for detecting if there’s any double quote within a given string.
3. Using the includes() Method
JavaScript’s includes() method, for strings, is an easy way to check for a double quote in a string.
javascript
let text = ‘Hello “JavaScript”‘;
if (text.includes(‘”‘)) {
console.log(“The string contains a double quote.”);
} else {
console.log(“The string does not contain a double quote.”);
}
Explanation:
- text.includes(‘”‘) returns true if there’s a double quote anywhere in the string, and false otherwise.
- This method is very readable. It is often preferred for checking specific characters.
4. Checking with Regular Expressions
Regular expressions (regex) are a powerful tool for pattern searching in text. You can use regex to find double quotes in a string as follows:
javascript
let text = ‘Sample “Text”‘;
if (/”/.test(text)) {
console.log(“The string contains a double quote.”);
} else {
console.log(“The string does not contain a double quote.”);
}
Explanation:
- The /”/.test(text) statement checks if the double quote ” appears in text.
- This method is great for pattern matching. It’s easy to extend if you need to check more complex patterns later.
5. Checking a Specific Character Position
To check if a character at a given index is a double quote, access it by index and compare it with ‘”‘.” Here’s an example:
javascript
let text = ‘He said, “Hello!”‘;
let index = 8;
if (text[index] === ‘”‘) {
console.log(`The character at index ${index} is a double quote.`);
} else {
console.log(`The character at index ${index} is not a double quote.`);
}
Explanation:
- text[index] gives you the character at the specified index (in this case, index 8).
- This approach is useful when you know the position of the character you want to verify.
6. Using charCodeAt() for Unicode Value Comparison
Another way to check if a character is a double quote is by comparing its Unicode value. The Unicode value for a double quote is 34.
javascript
let character = ‘”‘;
if (character.charCodeAt(0) === 34) {
console.log(“The character is a double quote.”);
} else {
console.log(“The character is not a double quote.”);
}
Explanation:
- charCodeAt(0) retrieves the Unicode value of the first character in character.
- This method is a bit advanced. But, it can help with character encoding.
Best Practices for Checking Double Quotes
- Choose the Right Method: For single-character checks, use ===. For broader searches in a string, includes() or indexOf() are good choices.
- Use Regex for Complex Patterns: For patterns with many characters, regex can save you time and code.
- Know When to Escape Characters: If you use double quotes in a double-quoted string, you must escape them. Example: let text = “He said, \”Hello!\””;.
Conclusion
In JavaScript, you can check if a character is a double quote in several ways. Each method is best for different contexts. These methods will help you handle double quotes. Use them for user input, JSON, or large text files.
FAQs
1. Why do I need to escape double quotes in JavaScript strings?
If you use double quotes to define a string, escape any double quotes inside it with a backslash (\”) to avoid errors.
2. What’s the Unicode value for a double quote?
The Unicode value for a double quote (“) is 34. This is useful if you’re checking characters by their Unicode values.
3. Which method is best for finding double quotes in a long text?
For large strings, use includes() or indexOf(). They are efficient and easy to read. They check if a string contains double quotes.